In this quick tutorial, I will walk you through a few quite interesting techniques exporting, encapsulating a group of functions into a single unit. How it will improve your understanding of node scope and exporting technique to build industry standard node_modules.
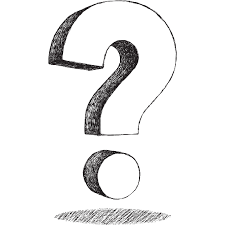
Before we get into the actual topic, we need to understand how we refer a function or a variable without using module.exports or exports. What are the cons if we follow them?
Lets we have js file named fun.js,
let data =10; console.log(data)
we will execute this code by calling/including this file in another file called index.js .
require('./fun')
Run code
> node index // output is : 10
So, here you can observe, that we refer a file which contains a variable “data” and while it is a part of the global scope of the program. As a good programming practice, we should avoid polluting global scope using variables. Reason,
- If it is in global scope , it stays till complete execution whole program.
- If we follow same approach for a big enterprise application, memory utilization of program would increase drastically.
- There might be a chance override the existing variable ,if you don’t have trace of variables used.
Above code could be written in below way as well,
function fun(data){ console.log(data) }
If we execute the same code,
require('./fun') fun(10)
Run code
> node index // output is : 10
You can observe the output remains same. But we have extra advantage, data variable won’t be available in global scope. It scope is in fun function. We are not polluting global scope .
Functions are small unit of program ,which hold responsibility for a particular work. There might be more than one similar function as per their nature of work or type . As an example , you can think of set of function doing some type of arithmetic calculation. To bundle them together into a single unit we use module.exports or exports .
module is a plain JavaScript object with an exports property. exports is a plain JavaScript variable , which is a set of module.exports .
- You can consider exports is short hand declaration of module.exports.
- You can use both in same file.
module.exports.add = function(a,b){ return a+b; } exports.stringLength = function(data){ return data.length; }
Module referred in index.js as below,
const {add} =require('./codescrap') const {stringLength} =require('./codescrap') console.log('add : ',add(6,7)); console.log('String Length : ',stringLength('Demo'));
Please check the code. you will be able to relate the similarity. Like this we can bundle functions in a class and export those using module.exports or exports.
Happy reading. Thanks for visiting.