Intro
Async & Await is introduced in ES6. It is very important to learn to make our effective and simple. It is a five minute tutorial with an example to make you aware of both. Quick and short explanation.
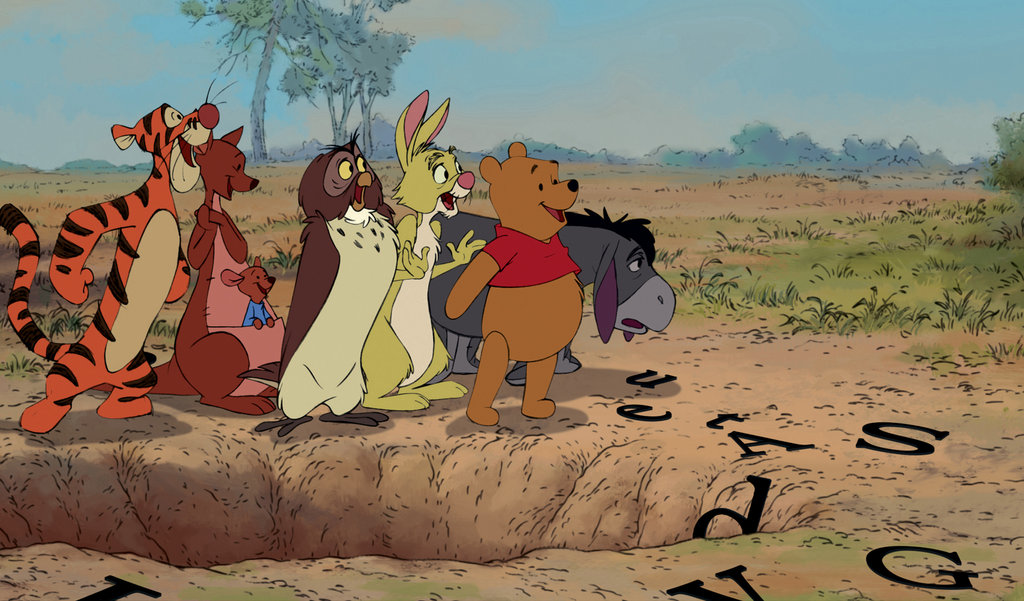
Async :
Let’s talk about async.
- It is placed before a function
- If you place async before a function, it will return a promise.
Let’s understand the above points,
// Async examples- it is placed before the function defination async function testCall(){ return 5; } // call the function console.log(testCall());
The output would be a Promise
Promise { 5 }
To resolve this Promise issue we need to write a promise handler code.
Above function call could be written in a way, as demonstrated below
//resolve promise testCall().then(function(result) { console.log(`result is ${result}`); }).catch((e) => { console.log(e); })
Async function with await makes the asynchronous function to synchronous function which allows you to do some operation on the returned value.
Await :
The keyword await tells the program to wait till the promise resolves and value is returned to the called function.
Syntax :
let value =await promise;
Note: Await works inside the async function only. i.e. the function inside which await is used, should carry async as a prefix. As demonstrated below,
testCall().then( async function(result) { let computeValue = await promise; }).catch((e) => { console.log(e);})
People generally make a mistake or got confused to decide which function should be defined as async.
Example code is :
// It come with Async only. It can't be used alone. // await only be used inside the async function async function callAwait() { let finaldata = await testCall console.log('final data', finaldata); } // execute async function - output : 5 callAwait() // Below code will throw error ,because await is used outside of async function // Error will be SyntaxError: await is only valid in async function let data = await testCall()
The same thing can be done using “Promise” in javascript. We will cover it in our next post.
Please feel free to post comments and suggestions.