This post is about getting familiar with the few of the Gulp operations and FTP deployment to a web server using it. As a developer, we have some challenges to manage the process of development and deployment to a different server. There are few of the techniques in Gulp, which can handle your hectic tasks pretty easily and make our life easy in the developments.
Old Approaches :
When we try to develop the new application or update a legacy application, we need to update the latest bunch of files in the remote server. One Application might have a different server like dev, integration, staging, pre, production etc.
Let’s I have five servers for an application XYZ, I will develop a code, deploy it to Dev and test. Then push one other server after approvals. It is a boring and non-productive task by using an old-school technique like tools or drag and drop option using tools.
I prefer to do it by using some command line technique, which will some minutes of my life. Some of the legacy application server using FTP connection to send the file and deploy it.
Note: I might note the best approach. But it solves some of the problems we face while development and deployment.
Gulp Installation:
Per-requisite for this you need to have Node.js installed in your machine. I am assuming , it is these in you system already . If it isn’t , Please download and install it. Please follow the link. click
Please open command line and type the below command for global installation, so that gulp CLI would be for direct use through command line.
npm install -g gulp
It is a popular build system for Node.js to enhance and automate the workflow. It uses stream for data transformation and managing the data from any source.
Project Set up
To try out below steps you need a FTP server credentials for deploy it . If you don’t need to worry , this knowledge will help you in your future works. Also you will be learning simple techniques for gulp command to placing file other folders etc.
We will be going through below tasks to learn simple tricks of gulp,
- Install Node.js and Gulp
- Create package.json to initialise the
NPM project set up:
Initialise NPM in the project folder, by typing below command,
npm init
It will ask for some information and then “yes” to finalise the package.json.
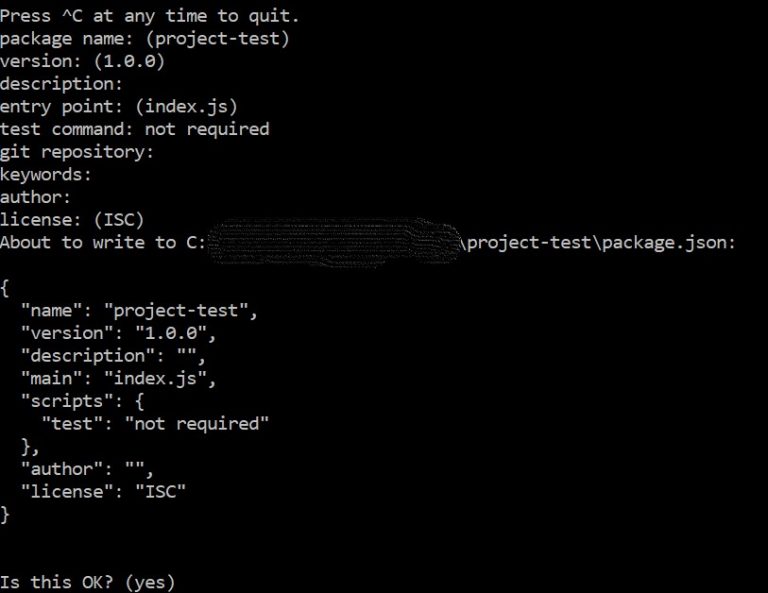
We need dependancies like gulp, gulp-util,vinyl-ftp to be installed. execute below command to install it.
npm install --save-dev gulp gulp-util vinyl-ftp
gulp-util modules help gulp for logging related actions. vinyl-ftp helps in FTP related actions.
Let’s go for creating gulpfile.js in the root folder of your project.
gulpfile.js
Let’ understand gulp tasks and how to write them,
Include the gulp module to gulpfile.js by below command.
var gulp=require('gulp')
Write the first task. Tasks will have below structure,
gulp.task('name',function(){ //task implementation code })
Sample code for copy task is
gulp.task('copy', function() { gutil.log('Copy started ') gulp.src('index.html').pipe(gulp.dest('webdemo')); gulp.src('webdemo/*').pipe(gulp.dest('build')); })
Here gulp is an object and task is a method of it. task accepts two methods.
- The name of the task
- Callback function (it can be arrayed other function calls
Some important methods of the gulp object,
- src: name of the file or folder paths to access the file and use it as an input.
- pipe: It takes the output of the previous command that executed and transfers it to the next command as an input.
- dest: Accepts the input data from the pipe command and write it to the destination folder.
to run the above task use command,
gulp copy
Above command will copy the index.html of parent project folder to webdemo. Then, the second one transfers all the files from the webdemo to the build folder.
Generally, patterns for file or folders would be like webdemo/*.js or build/css/*.css to copy particular patterns.
var gulp = require('gulp'); var gutil = require('gulp-util'); var ftp = require('vinyl-ftp') /** Configuration **/ var user = process.env.FTP_USERNAME var password = process.env.FTP_PASSWORD var host = 'host.com' var port = 21 var remoteFolder = '/public_html/' gulp.task('copy', function() { gutil.log('Copying started') gulp.src('index.html').pipe(gulp.dest('webdemo')); gulp.src('webdemo/*').pipe(gulp.dest('build')); }) // helper function to build an FTP connection based on our configuration function getFtpConnection() { return ftp.create({ host: host, port: port, user: user, password: password, parallel: 5, log: gutil.log, }) } /** * Deploy task. * Copies the new files to the server * * Set the FTP_USERNAME and FTP_PASSWORD to env variable * or easyway you can set it in the program it self */ gulp.task('ftp-deploy', function() { var conn = getFtpConnection() return gulp .src('index.html', { base: '.', buffer: false }) .pipe(conn.newer(remoteFolder)) .pipe(conn.dest(remoteFolder)) })
In the above program, we are creating an FTP connection using vinyl-ftp to access the FTP server.
You need to pass hostname of the server (abc.com or 192.158.1.201), credentials of the server (username and password ). We are using process object to get the credentials from env variable. FTP_USERNAME and FTP_PASSWORD are used as env variable for credentials.
You can hard code it in the program. We are using port no 21 for default FTP connection.
remoteFolder is holding the folder location of the server where the files need to be copied.
Running Command:
To set the env variable in command line ,
set FTP_USERNAME=username set FTP_PASSWORD=password123
Run deployment task is ;
gulp ftp-deploy

Please feel free to comment and suggest.