Node JS and JavaScript Intro:
With the introduction of Node Js, a new era has been started for Javascript. A massive and continuous growth in JavaScript implementation and concepts is on. It has taken become one of the major languages in web design and development.
Node JS
Node.js is a very powerful JavaScript-based framework/platform built on Google Chrome’s JavaScript V8 Engine. It is used to develop I/O intensive web applications like video streaming sites, single-page applications, web etc.
Data Structure using JavaScript:
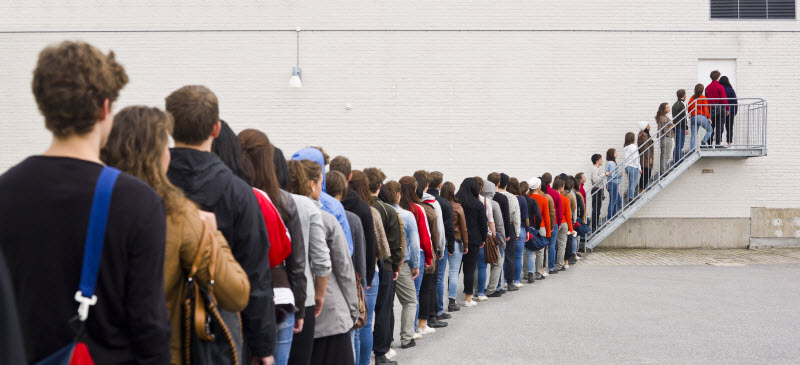
Queue
The Queue is a particular data structure in which data is arranged in particular format. That Format is called FIFO (First In First Out).
FIFO means whoever comes First into the storage system will leave first from the system. An example is Queue in ticket counter.
The person who comes first to the ticket counter queue will get the ticket first.
In an industry like coca cola, the machine fills the bottle which comes first for filling then next bottle. Like this queue concept implemented everywhere.
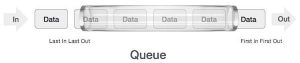
Declare Queue Module
function Queue(){ // this is where all the varibales are declared.......................... var head =null; //This is the pointer for head section of the Queue.where item // enters to the queue.means enqueue operation var tail =null; // This is the pointer for tail section of the Queue. Where only // Dequeue operation takes place. First enter item leaves first //from this end. var count=0; // keeps the count of number of elements in the Queue //Below are the methods related to this Queue................. //returns the no of items in the Queue this.getCount=function(){ return count; } }
Enqueue
It adds element/item to the front of the Queue. It is used for insertion to the Queue. The counter is increased by one in every enqueue operation.
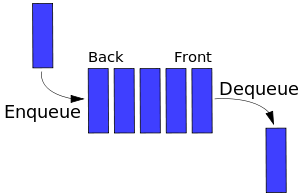
Enqueue Operation
this.enqueue=function(item){ var node ={ data : item, next: head } // if it is first element in the queue so tail should be pointed to first arrival if(head==null){ tail=node; } // pointing head to the top of the queue head=node; //increase the counter of the queue count++; }
Dequeue
This operation is used for removing the element from the tail end of the Queue. On every dequeue operation counter is decreased by one.
Dequeue Operation
this.dequeue=function(){ if(tail==null){ return null; }else{ var currItem=head; var prevItem=null; /* We need to iterate through the queue items and store the previous items in prevItem for assigning to tail when we read the last item. tail Item of the queue stores 'null' in the referrance setion. using this identifier we will identify the last element */ while(currItem.next){ prevItem=currItem; currItem=currItem.next; } //if the queue contains more the one element we need to dereferrance the last element means we need to assign 'null' to tail element after removal of element if(count ‹1){ // assign null of dereferrancing the item before tail element prevItem.next=null; // tail pointing to the previous node tail=prevItem }{ // if it contains a single element. Queue is empty after removal head=null; tail=null; } // decreasing the element count count--; //if you want to see the remove element put return statement like below. otherwise return is not required return currItem; } }
peekAt
This operation is used for seeing/searching desired element by index. We need to pass valid index for search, otherwise, it will return null.
peekAt Operation
this.peekAt=function(itemIndex){ // check the length of the queue and index asked for if(itemIndex‹count && itemIndex›-1){ var currItem=head; for (var i = 0; i ‹ itemIndex; i++) { currItem=currItem.next; } return currItem.data; }else{ // if condition fails. return 'null' for invalid request return null; } }
diaplayAll
This operation is a customized way to see the elements in the Queue.
displayAll Operation
this.displayAll=function(){ var ar=[]; var currItem=head; if(count===0){ return null; }else{ for (var i = 0; i ‹ count; i++) { ar.push(currItem.data); currItem=currItem.next; } } return ar; } }
You can get the whole code from the Github repo. Below is the link:
https://github.com/sahajahanAlli/queue-example
- Navigate to the folder(queue-example) using command prompt/windows powershell
- Type command “npm install”. It will install node modules.
- Type command “npm start”. It will show the output.
Output
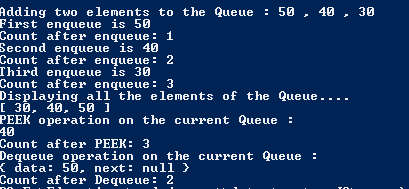
Please feel free to share your comments.
2 comments
I’ve been browsing online more than three hours today, yet I never found any interesting article like yours. It’s pretty worth enough for me. In my view, if all site owners and bloggers made good content as you did, the internet will be a lot more useful than ever before.
Thank you very much. Keep visiting, we will publish a lot more content like this.